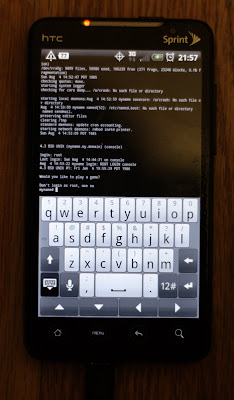
In order to make this process easier on my phone, I used a rooted firmware. It will take some more effort to get this to work as a packaged application.
Setting up the development environment.
To get started, you'll need a working native C compiler for Android. After a lot of trial and error, I ended up discovering what I had to do. I used an amd64 architecture version of Debian GNU/Linux, Ubuntu works the same way. Using a Linux host to compile the code if not essential will make your life a lot easier. Follow the official instructions to download the source to Android using git, and then build it.
Along with doing that, I tested this on the Android emulator, which is a part of what you just downloaded and built, under out/host/linux-x86/bin. Put that directory in your path, and run the "android" command, create a virtual platform, and boot it. From the command line, if you built a virtual device named "Android21", for example, you'll want to run "emulator -avd Android21 -shell" so that you can get a shell on the virtual device. To copy files over to the image, the easiest method that I've found is to shut down the emulator, mount the virtual sdcard image (for these examples, I'm using "Android21" as the virtual device name):
$ sudo mount -o loop ~/.android/avd/Android21.avd/sdcard.img /mnt
$ sudo cp whatever /mnt
$ sudo umount /mnt
In addition, you will want the "agcc" script to make it easier to compile things. Download it from here. I modified my copy to use gcc-4.4.0 instead of gcc-4.2.1. You will need to add the location of arm-eabi-gcc to your path, and change all references of "4.2.1" in the agcc script to "4.4.0" and add that to your path. arm-eabi-gcc can be found under the prebuilt/linux-x86/toolchain/arm-eabi-4.4.0/bin path of where you built the android sources.
Fixing the Android SDK and SIMH makefile
The current version of the Android libc (bionic) headers has a problem compiling when bionic/libc/kernel/arch-arm/asm/byteorder.h is included. In order to make the file compile, comment out these lines, lines 22-27 in my copy:
/*#ifndef __thumb__
* if (!__builtin_constant_p(x)) {
*
* asm ("eor\t%0, %1, %1, ror #16" : "=r" (t) : "r" (x));
* } else
*#endif */
Once you do that, you will need to need to download the SIMH sources. Unpack them, and modify the the makefile in the top directory, to make the following changes:
On line 12, remove -lrt from OS_CCDEFS, so that it reads:
OS_CCDEFS = -lm -D_GNU_SOURCE
Change all references of "gcc" to "agcc".
Only some of the simulators actually compile, and I haven't tried to compile network support for any of it. In order to get network support, you would need to compile libpcap as well. For now, that is left as an exercise for the reader. :)
After those changes, I just did make vax vax780 to build the MicroVAX 3900 and VAX-11/780 simulators, which can be copied over to your phone or emulator. You will probably get a bunch of warnings about the use of variable-size enums versus 32-bit enums. They seem to be harmless, and I'm pretty sure that you can ignore those warnings.
If you're too lazy to compile it
If you don't feel like spending the time to set up a development environment, and compile SIMH by yourself, I have a pre-compiled version for Android available. I used SIMH v3.8-1, which is the newest release as of this posting. You can get a pre-compiled copy of the Android emulator to test on from the pre-compiled Android SDK. After you download and unpack that, you will need to put the tools directory inside the sdk into your path.
Preparing your phone and copying things over
You will need a rooted phone to make this work easily. Rooting your phone is left as an exercise for the reader. Once you have root permissions, you will need to USB debugging on your phone. On my Evo, it's under Settings -> Applications -> Development -> USB debugging.
You will need an application that will work as a terminal emulator on your phone. You can use "adb shell" from the Android SDK, which will give you a shell from your phone on your computer. To run completely hosted from the phone, use something like ConnectBot.
From this point you can copy files necessary for the emulator to your phone. I copied the necessary files all to my phone's SD card:
$ adb shell mkdir /sdcard/simh
$ adb push BIN/vax /sdcard/simh
$ adb push VAX/ka655x.bin /sdcard/simh
...
Now, use adb shell to do a few things on the phone itself. Pay attention to what your phone is saying, as the first time you try to "su" on your phone, it may pop up a dialog asking if this is ok. Whether or not you see that will depend on exactly how you rooted your phone. If "adb shell" gives you a "#" prompt straight away, you don't need to use su, as you're already root.
$ adb shell
$ su -
# mkdir /data/simh
# cat /sdcard/simh/vax
# chmod 755 /sdcard/simh/vax
It is necessary to put any executables on the internal storage (eg in /data/simh like I did), because you cannot directly execute binaries from the sdcard, at least in Android 2.1.
Running a SIMH emulator
Once you've done that, as root on the phone, do something like:
# cd /sdcard/simh
# /data/simh/vax
And then just use SIMH as you normally would.
Connectbot allows for multiple simultaneous sessions, so you could do "set console telnet=2300" inside the emulator, and then open another telnet session in connectbot to 127.0.0.1:2300 to connect to a separate console. Connectbot simulates "screen" as a terminal emulator, which seems to do an adequate VT100 emulation for most things. The most I've tested it so far it running /usr/games/worms from 4.3BSD, after "setenv TERM vt100". If you have your device on a WiFi network, you could even use another machine to telnet into SIMH and be the console, or another emulated serial terminal on the system.
That's it! If you don't know what to do from here, take a look around the SIMH site, you can run things like ancient versions of UNIX, OpenVMS through HP's hobbyist program, NetBSD, or play with the other emulators and other OSes. Where you go from here is up to you.
EDIT Aug 8, 2010:
I forgot to add the pictures that I have taken of this. Check them out on flickr. I also now added the picture to the top.
4 comments:
Thanks for the post! I've wanted a VAX in my pocket for quite awhile now. There was a Purchaseable version on SIMH on the Android market, but I didn't want to pay the $5 for it. Was looking at figuring out the toolchain setup for it, but you beat me to it. Thanks!
I'd love to have a VAX in my pocket or even on my desk! And I'd even pay $5... but rooting I'm a little squeamish about.
I've recently been given a Samsung Galaxy Tab (lucky me!) and once again, am indebted to you for this useful tutorial. The byteorder.h issue can be kludged by replacing 'asm' with '__asm' - but I got lots of warnings about variable-sized enums vs. 32-bit enums, and later, pdp10 aborts with undefined references. Most of the simulators compile.
pdp10 compiles if you switch on optimization, or if you specify any -O flags.
Post a Comment